Tricky C# interview questions for Senior developers
Often the C# interview questions for Senior Position revolve around different OOPS principles like SOLID, DRY, KISS and architectural patterns like layered architecture or CQRS pattern, design patterns, and system design questions. But there are times when you can feel trapped if the same monotonous questions is asked from a different perspective which I use in my interviews.
Below are some of the tricky questions I ask to know if the candidates understands the underlying concept or is just a rhetoric.
Trap 1: Tell me, what do you understand by Open Closed Principle?
Often the candidate explains that its a principle in which a “class is opened for extension but closed for modification”. Yes, that’s right and that’s exactly what is written on internet too.
Tricky question: Tell me, how does Open closed principle gets violated in code?
This is where I have seen most of the candidate’s explanation falling apart.
Even if you have learned practiced all of the SOLID principles, you must know what kind of code violates of those principles.
Ask yourself: How will I prove what kind of code violates each of those principles.
Trap 2: What is the difference between read-only and const keyword?
Answer: Read-only is a runtime constant and const is a compile time constant. You can assign the read-only value at the time of declaration or in a constructor.
Tricky question: So, if I use readonly keyword with a list, will it become readonly?
public readonly List<string> _productNames;
Answer: No.
Follow up question: Why is that? How can I create a readonly list?
Trap 3: Make the below class immutable.
public class Manager
{
public string Id {get; set; }
public string Name {get; set;}
public Manager(string id, string name) {
Id = id;
Name = name;
}
}
Often candidate know only the rhetoric definition of immutability and often relate it with strings only. Immutability is a concept that can be applied to all the types in any programming languages.
The answer is simple: Immutability means that anything that gets set once cannot be modified. In this case, you can create a manager instance and set its properties via constructor but then you can later change the Id or Name too (if you want) coz they have public setter. So you just need to remove “set” part from the properties to make the class immutable. This way, once an instance is created, it cannot be modified. You can create a new instance each time you want to change something in existing instance.
Trap 4: How will you remove tight coupling between two classes?
Answer: By introducing interfaces between them and making use of dependency injection.
Right!
Trick question: If a class constructor gets over injected by dependencies, which Solid principle is getting violated here?
(Comment your answer :) )
Trap 5: How memory gets managed in C#?
Answer: There are 2 types of memories. Heap and Stack. Value types get stored on stack and reference types get stored on heap.
Right!
Trick question: In the below code, where would _orderId and _order get stored in the memory?
public class A
{
public int _orderId = 0;
public Order _order = new Order();
}
Often the candidates tell that _orderId will get stored on stack coz its a value type and _order will get stored on heap coz its a reference type.
But its wrong! Both will get stored on heap. Even if _orderId is a value type but it is within a reference type which is a class. And as long as the class is alive, its class level members will be alive too.
Question 1: If a class has three constructors — static, protected and public, what will be the order of execution for these constructors?
This is a trick question. Candidates often tell me that its static, then protected and then public will get executed. However, that is wrong. A class cannot have protected and public constructors at same time. You can have a static constructor and public or protected or internal constructor but not both.
Question 2 : Follow the below code snippet
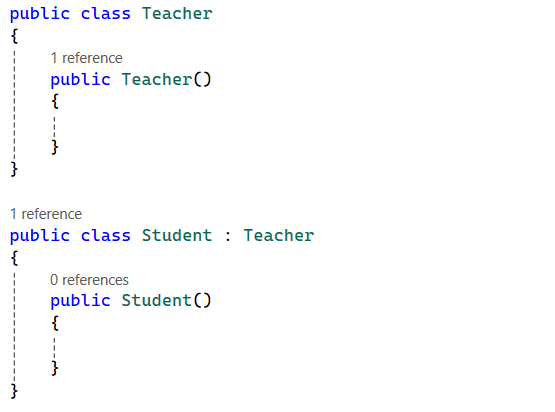
Tell me the order in which constructors will get executed for below code snippet:
Student student = new Student();
Teacher teacher = new Student();
Teacher teacher = new Teacher();
Let me know your answer in comments :)
Question 3 : What is the difference between overriding and shadowing a method?
Overriding can only be done if the base class method has been marked as virtual, matches the same return type, parameter(s) and order of parameter(s).
However, shadowing can be done on any method with same name in base class. It does not require virtual keyword to override it.
Good so far?
Now tell me if below code snipped is valid and if it is then where would you use it?
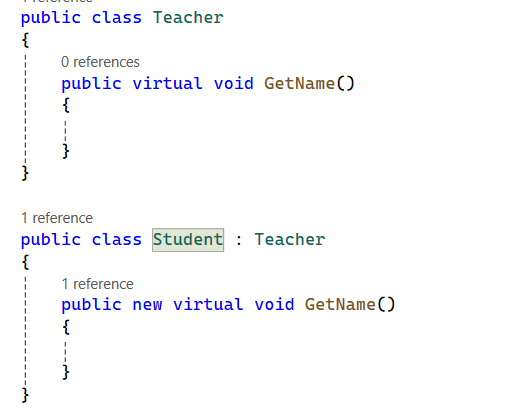
Question 4: What’s the difference between signed and unsigned integers? Where would you use which one?
Question 5: What’s the difference between Abstraction and Encapsulation?
Answer: This is the most basic question and I have hardly seen any candidate explaining this clearly to me.
Abstraction is a principle used in every day modern life as well as in coding too. Anytime, we want to hide the complexities of a task, we are abstracting it. A car’s engine is hidden under its bonnet. Its Abstraction!
You method GetDataFromTheServerInAntartica() is making a call to the server in Antartcia to get the data is an abstraction! Why? because its hiding the complexity of making a call to server, waiting for data, extracting the data and finally send to the user.
Now when it comes to define Encapsulation, often I hear from candidates is — It’s used for data hiding.
Wait a minute! Hiding again? Wasn’t that the same in Abstraction?
And the explanation topples. Encapsulation is the principle of creating logical boundaries around your code so that no new developer calls (by using new keyword) and manipulates the data he is not supposed to. And how do we do it? By using proper access modifiers!
Here you are hiding again but its not same as abstraction.
Question 5 : Why do we need Encapsulation at all? What if I declare all the methods and classes as public in my very small application? What is the problem with that?
Answer: This one too is difficult to answer if you have not understood the concept of Encapsulation.
Often candidates say — Its a bad practice. Yes, but why?
Going back to the previous answer, we have Encapsulation so that no developer can call or manipulate the data of a class he is not supposed to. Right?
Fair! But why? What if he does?
Then that would screw the state of the application. Imagine a user’s data is getting saved into another user’s state. How chaotic would that be!
So in short, we are trying to save/manage the state of the application by using proper Encapsulation!
If you like my content, please leave a thumb up. Next article will provide simple yet tricky system design interview questions too which I ask in my interviews.
댓글